· Tutorials · 5 min read
Docker for beginners - Part 5 - Docker Compose
Docker Compose is a tool for running multi-container applications. It defines the services that make up your app in docker-compose.yml so they can be run together in an isolated environment.
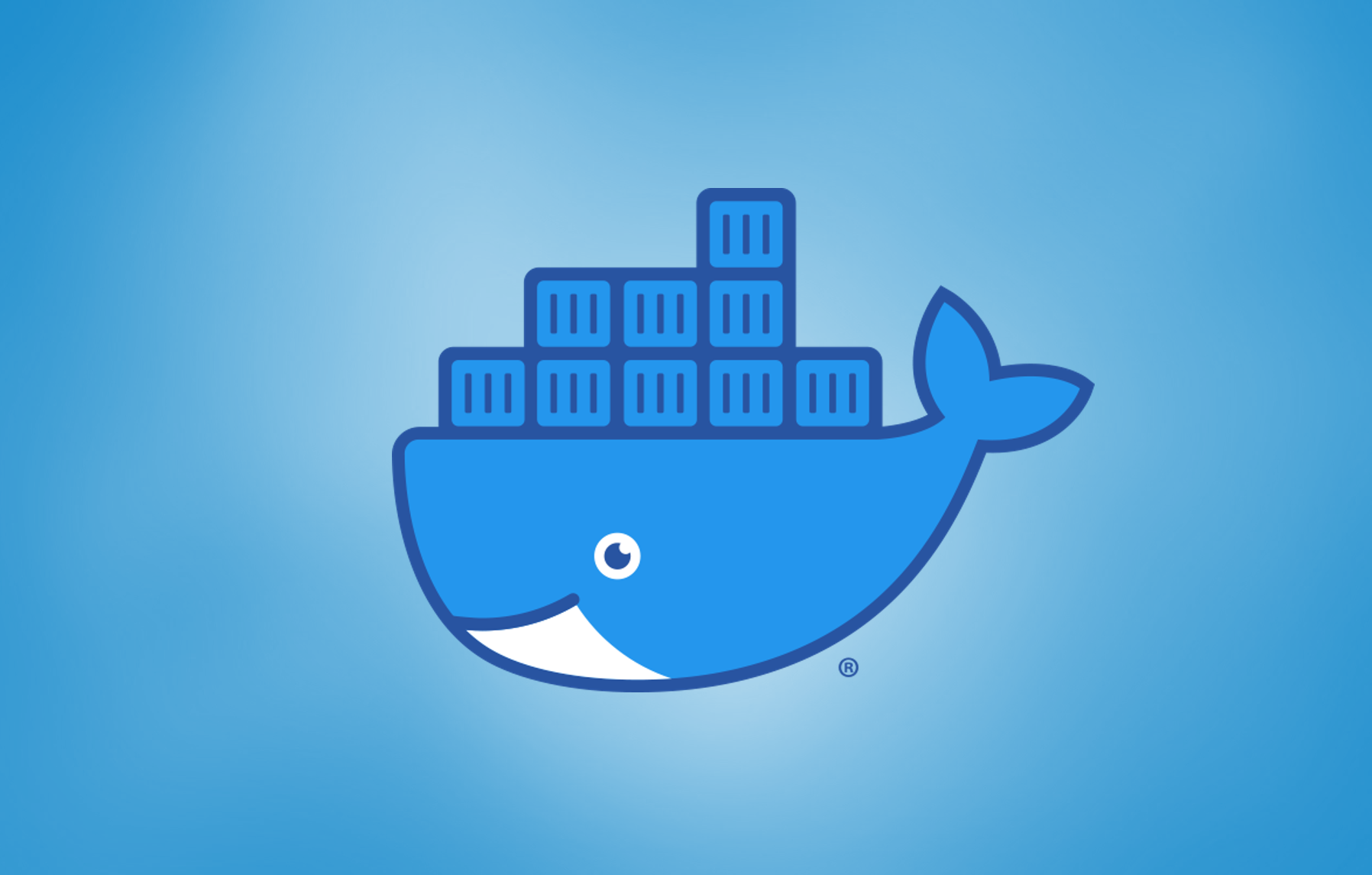
Series Overview
- Part 1 - Docker for beginners - Introduction and Installation
- Part 2 - Docker for beginners - Docker Images and Containers
- Part 3 - Docker for beginners - Docker CLI
- Part 4 - Docker for beginners - Dockerfile
- Part 5 - Docker for beginners - Docker Compose (You are here)
Docker Compose
Docker Compose is a tool for running multi-container applications. It defines the services that make up your app in docker-compose.yml so they can be run together in an isolated environment.
In simple terms, Docker Compose is a tool that allows you to run multiple Docker containers at the same time, while coordinating the containers to work together.
Docker Compose comes installed by default with Docker Desktop.
Why exactly do we need Docker Compose?
Let’s say you have a web application that uses a database. You will need to run two containers, one for the web application and one for the database (A Container can indeed run a database!). You will also need to configure the web application to connect to the database. You can do this by using environment variables. But still this involves a lot of manual work. You will need to run the database container first, then you will need to run the web application container and set the environment variables for the web application to connect to the database. We need a way to automate this process.
This is where Docker Compose comes in handy. Docker Compose allows you to automate the process of running multiple containers and configuring them to work together. It does this by using a YAML file called docker-compose.yml
. This file contains the configuration for all the containers that you want to run and how they should work together. You could just run the docker-compose up
command and Docker Compose will take care of the rest.
If you can’t keep up with the explanation, that’s perfectly alright, we’ll understand by doing an example.
Docker Compose Example
Let’s quickly setup a simple ASP.NET Core app with a SQL Server database using Docker Compose.
Step 1 - Create a new ASP.NET Core app
Let’s create a ASP.NET Core app by hand, especially for those who don’t have .NET installed on their machine.
Create a new folder called docker-compose-example
and create a new file called Program.cs
in the folder.
Add the following code to Program.cs
:
var builder = WebApplication.CreateBuilder(args);
var app = builder.Build();
app.MapGet("/", () => "Hello World!");
app.Run();
Create a new file called DockerComposeExample.csproj
in the same folder and add the following code to it:
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net7.0</TargetFramework>
<ImplicitUsings>enable</ImplicitUsings>
</PropertyGroup>
</Project>
Create a new file called Dockerfile
in the folder and add the following code to it:
FROM mcr.microsoft.com/dotnet/sdk:7.0 AS build
ENV ASPNETCORE_URLS=http://+:5000
WORKDIR /src
COPY ["DockerComposeExample.csproj", ""]
RUN dotnet restore "./DockerComposeExample.csproj"
COPY . .
WORKDIR "/src/."
RUN dotnet build "DockerComposeExample.csproj" -c Release -o /app/build
RUN dotnet publish "DockerComposeExample.csproj" -c Release -o /app/publish
WORKDIR /app/publish
ENTRYPOINT ["dotnet", "DockerComposeExample.dll"]
Finally, create a new file called docker-compose.yml
in the folder and add the following code to it:
version: '3.9'
services:
db:
image: mcr.microsoft.com/mssql/server:2022-latest
ports:
- 8081:1433
environment:
ACCEPT_EULA: "Y"
SA_PASSWORD: "Password123"
web:
build: .
ports:
- 8080:5000
depends_on:
- db
Step 2 - Run the app
Run the following command to build the app:
docker-compose build
Run the following command to start the app:
docker-compose up -d
Run the following command to view the logs:
docker-compose logs -f
Open your browser and navigate to http://localhost:8080. You should see the following:
If you have SQL Server Management Studio installed, you can connect to the database using the following details:
- Server: localhost,8081
- Username: sa
- Password: Password123
- SQL Server Authentication
Though we have not configured the web application to connect to the database, we have configured the database container to run along with the web application container, using Docker Compose.
You can see the containers running, in the Docker Desktop app:
Step 3 - Stop the app
Run the following command to stop the app:
docker-compose down
You can also stop the containers from the Docker Desktop app.
Wrapping up
We have managed to run a ASP.NET Core app with a SQL Server database, without installing .NET or SQL Server on our machine and configured them to run side by side using Docker Compose. We have just scratched the surface of Docker Compose. A lot of advanced concepts like Docker Compose networks, volumes, etc. are not covered here, as the focus of this tutorial is to provide a basic understanding of Docker Compose. Some of the concepts like Docker Compose networks, volumes, etc. will be covered in upcoming series in context of microservices.
This tutorial is just meant to give you a basic understanding of Docker Compose in general. If you want to learn more about Docker Compose, I recommend you to check out the official documentation. But still I would recommend you to learn Docker Compose in context of microservices, as that is where Docker Compose shines.
Hope you enjoyed this series and gained a solid understanding of Docker. If you have any questions, feel free to reach out to me on Twitter or LinkedIn.