· Tutorials · 6 min read
Docker for beginners - Part 4 - Dockerfile
Dockerfile is a text file that contains all the commands to build a Docker image. In this tutorial, you will learn about Dockerfile.
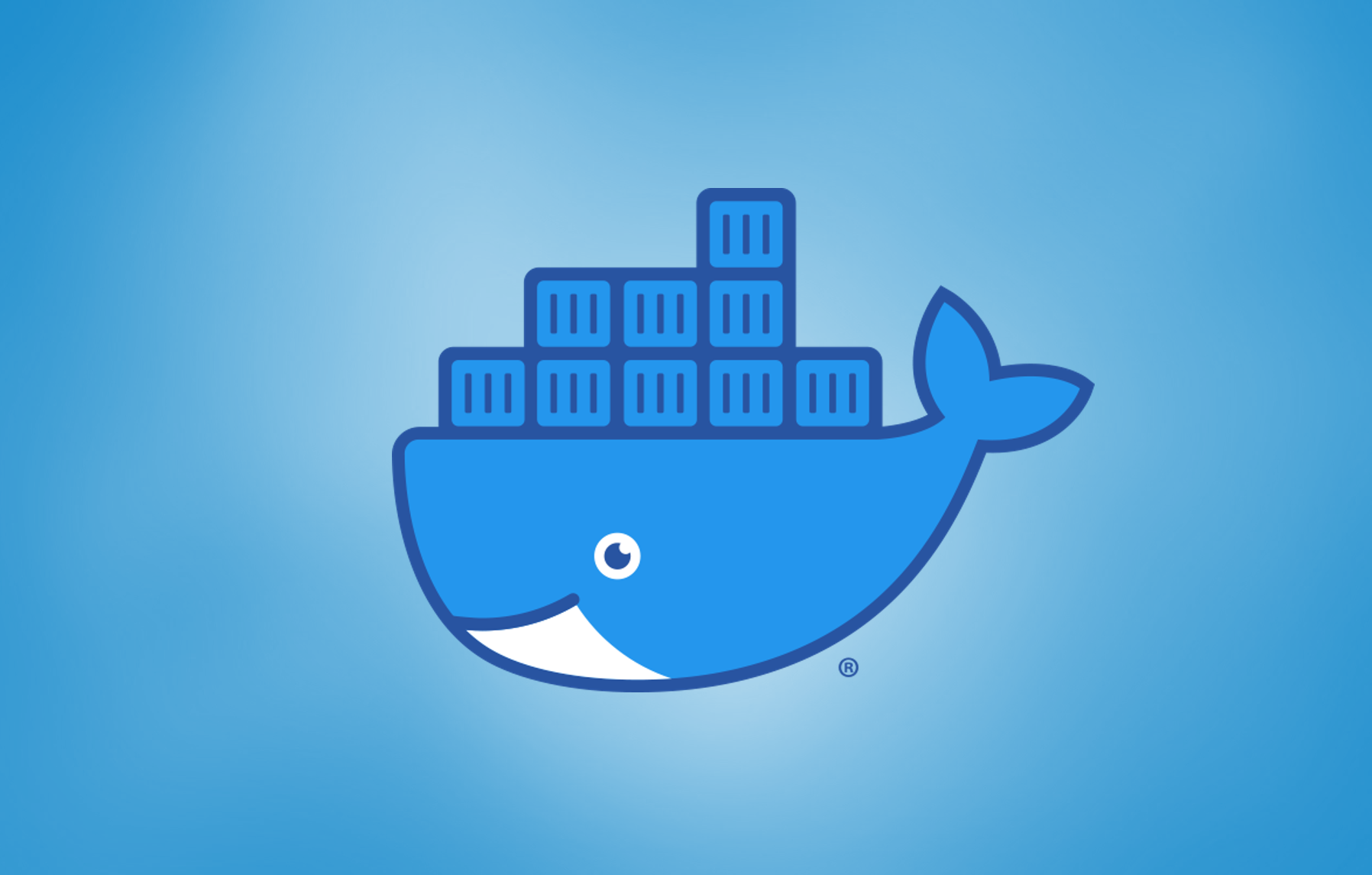
Series Overview
- Part 1 - Docker for beginners - Introduction and Installation
- Part 2 - Docker for beginners - Docker Images and Containers
- Part 3 - Docker for beginners - Docker CLI
- Part 4 - Docker for beginners - Dockerfile (You are here)
- Part 5 - Docker for beginners - Docker Compose
What is Dockerfile?
Dockerfile is a text file that contains instructions to build a Docker image.
As a developer, we may have built an application and to use docker, we need to somehow package it into a container image, so that we can run it on any machine as a container. Dockerfile is just a text file that contains instructions to automatically build a Docker image. In this section, we start with a simple Dockerfile and then we will learn about some of the most commonly used Dockerfile instructions.
Dockerfile Example
Let’s start by exploring a simple Dockerfile. In your local machine, open a folder and create a file named Dockerfile
and add the following content to it.
FROM nginx:1.19.10
COPY index.html /usr/share/nginx/html
Then create a file named index.html
in the same folder and add the following content to it.
<h1>Hello World!</h1>
The above Dockerfile contains two instructions.
FROM
instruction is used to specify the base image. In this case, we are using thenginx:1.19.10
Docker image as the base image.COPY
instruction is used to copy theindex.html
file from the current directory to the/usr/share/nginx/html
directory in the Docker image.
I know it’s bit confusing about what’s happening, but don’t worry, I will explain it in detail in the next section. For now, let’s try to build a Docker image from the above Dockerfile.
Building Docker Image
To build the Docker image, open the terminal and navigate to the directory where the Dockerfile
is present and run the following command.
docker build -t my-image .
The above command will build the Docker image with the name my-image
from the Dockerfile
in the current directory.
Running Docker Container
To run the Docker container, run the following command.
docker run -d --name my-container my-image
The above command will run the Docker container with the name my-container
from the my-image
Docker image in the background.
Accessing the Application
To access the application, open the browser and navigate to http://localhost:80
. You should see the following output.
So what’s happening?
Let’s try to understand what’s happening in the above example.
We are actually running a web server to serve our Hello World html page. The web server is running inside a Docker container. The Docker container is running from a Docker image. The Docker image is built from a Dockerfile. The Dockerfile contains instructions to build the Docker image.
The Dockerfile starts with a
FROM
instruction. TheFROM
instruction is used to specify the base image. In this case, we are using thenginx:1.19.10
Docker image as the base image. Usually we use a base image that contains the operating system and other dependencies required to run our application. In this case, thenginx:1.19.10
Docker image contains thenginx
web server.With base image in place, we can now add our application code (Here, our Hello World html page) to the Docker image. The
COPY
instruction is used to copy theindex.html
file from the current directory to the/usr/share/nginx/html
directory in the Docker image. The/usr/share/nginx/html
directory is the default directory where thenginx
web server looks for the html files.Once we build and run the Docker image, the
nginx
web server will be started and it will serve theindex.html
file from the/usr/share/nginx/html
directory.By default, the
nginx
web server listens on port80
. Since we haven’t specified any port mapping, the Docker container will be accessible on port80
of the Docker host. In this case, the Docker host is our local machine. So we can access the Docker container on port80
of our local machine, by navigating tohttp://localhost:80
in the browser.
This is just a simple example to get you started with Dockerfile. In the next section, we will learn about some of the most commonly used Dockerfile instructions.
Dockerfile Instructions
In this section, we will learn about some of the most commonly used Dockerfile instructions.
FROM
FROM
instruction is used to specify the base image. For example, to use the nginx:1.19.10
Docker image as the base image, we can use the following FROM
instruction.
FROM nginx:1.19.10
RUN
RUN
instruction is used to execute a command inside the Docker image. For example, to install curl
inside the Docker image, we can use the following RUN
instruction.
RUN apt-get update && apt-get install -y curl
COPY
COPY
instruction is used to copy files from the current directory to the Docker image. For example, to copy the index.html
file from the current directory to the /usr/share/nginx/html
directory in the Docker image, we can use the following COPY
instruction.
COPY index.html /usr/share/nginx/html
CMD
CMD
instruction is used to specify the command to run when the Docker container is started. For example, to start the nginx
web server when the Docker container is started, we can use the following CMD
instruction.
CMD ["nginx", "-g", "daemon off;"]
ENTRYPOINT
ENTRYPOINT
instruction is used to specify the command to run when the Docker container is started. For example, to start the nginx
web server when the Docker container is started, we can use the following ENTRYPOINT
instruction.
ENTRYPOINT ["nginx", "-g", "daemon off;"]
WORKDIR
WORKDIR
instruction is used to specify the working directory inside the Docker image. For example, to set the working directory to /usr/share/nginx/html
inside the Docker image, we can use the following WORKDIR
instruction.
WORKDIR /usr/share/nginx/html
ADD
ADD
instruction is used to copy files from the current directory to the Docker image. It is similar to the COPY
instruction, but it has some additional features. For example, to copy the index.html
file from the current directory to the /usr/share/nginx/html
directory in the Docker image, we can use the following ADD
instruction.
ADD index.html /usr/share/nginx/html
EXPOSE
EXPOSE
instruction is used to specify the port on which the application inside the Docker container is listening. For example, to specify that the application inside the Docker container is listening on port 80
, we can use the following EXPOSE
instruction.
EXPOSE 80
VOLUME
VOLUME
instruction is used to specify the volume inside the Docker image. For example, to specify the /var/log/nginx
directory as the volume inside the Docker image, we can use the following VOLUME
instruction.
VOLUME /var/log/nginx
ENV
ENV
instruction is used to specify the environment variables inside the Docker image. For example, to specify the NODE_ENV
environment variable with the value production
inside the Docker image, we can use the following ENV
instruction.
ENV NODE_ENV production
USER
USER
instruction is used to specify the user inside the Docker image. For example, to specify the root
user inside the Docker image, we can use the following USER
instruction.
USER root
ARG
ARG
instruction is used to specify the build arguments. For example, to specify the NODE_ENV
build argument with the value production
, we can use the following ARG
instruction.
ARG NODE_ENV=production
Wrapping Up
That’s all for this section. In this section, we learned about Dockerfile in depth. Don’t worry if you don’t remember all the instructions. You can always refer the Dockerfile reference to learn more about the Dockerfile instructions. In the next section, we will learn about Docker Compose.