· 1 min read
AutoReversing Migrations in FluentMigrator
Know how to automatically generate reverse migrations in FluentMigrator using the AutoReversingMigration class.
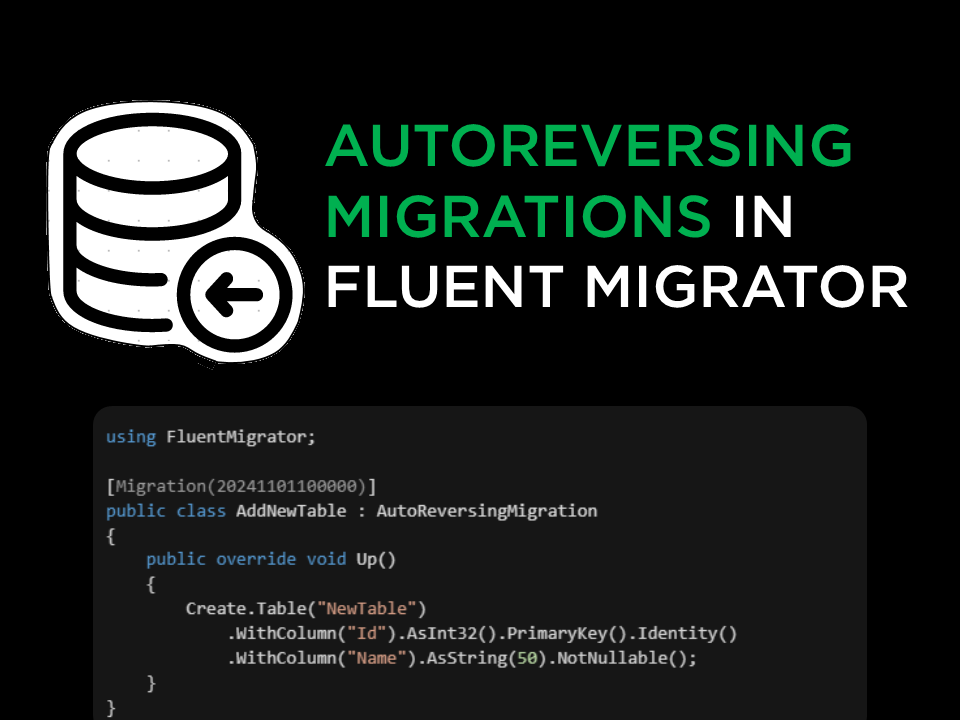
One interesting thing I found today in FluentMigrator while typing through IntelliSense is the AutoReversingMigration
class. This class is used to generate the reverse migration automatically. This is slightly different from the regular Migration
class.
The AutoReversingMigration
class doesn’t have a Down()
method. Instead, it automatically generates the reverse migration based on the Up()
method.
Here is an example of how to use the AutoReversingMigration
class.
using FluentMigrator;
[Migration(20241101100000)]
public class AddNewTable : AutoReversingMigration
{
public override void Up()
{
Create.Table("NewTable")
.WithColumn("Id").AsInt32().PrimaryKey().Identity()
.WithColumn("Name").AsString(50).NotNullable();
}
}
However, the AutoReversingMigration
class has some limitations compared to the regular Migration
class. For example, you can’t figure out the reverse migration for Execute.EmbeddedScript()
or Execute.Script()
, and you can’t use the AutoReversingMigration
class for those scenarios.
According to the FluentMigrator documentation, the only methods that are supported by the AutoReversingMigration
class are:
Create.Table()
Create.Column()
Create.ForeignKey()
Create.Index()
Create.Schema()
Rename.Table()
Rename.Column()
Delete.ForeignKey()
For other scenarios, you still have to use the regular Migration
class and implement the Down()
method manually.